When using the Cicode forms, the FormButton() can either be of 3
modes.
Button mode:
0 – Normal - callback function is called.
1 - OK -Form is closed, and all operator-entered data
is copied to buffers (specified by the other form functions).
FormRead() returns 0 (zero) to indicate a successful read. The
callback function specified in Fn is called. Note that this mode
destroys the form.
2 - Cancel - Form is closed and operator-entered data
is discarded. FormRead() returns with an error 299. The callback
function specified in Fn is called. Note that this mode destroys
the form.
The FormButton modes also have a built in Priority over which
button will get the focus.
When the Mode is set to 0 (or Normal), the button priority is
determined by the order that the button is defined in the Cicode,
regardless of the physical position on the form.
If there are an OK and a Cancel button in a form, then the default
selection will always be set to the OK button.
It may be possible to make the buttons both Normal (0) mode and
create custom callback functions, however, greater flexibility is
given by using a CiVBA MsgBox.
iResponse
= Msgbox ("Windows Title",MSGBOX TYPE, "Prompt Dialog")
For example:
Public
Function
AreYouSureCiVBA()
Dim
iResponse
As Integer
iResponse
= Msgbox ("Are You Sure You Want To
Continue?",257, "Are You Sure?")
If
iResponse
= 1 Then 'OK
AreYouSureCiVBA
= 1
Else
AreYouSureCiVBA
= 0 'CANCEL
End
If
End
Function
Will Display the following: and return 0 or 1 depending on which
option is chosen.
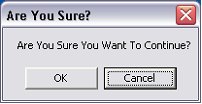
This CiVBA function can then even be called by a CiCode function,
to directly replace the CiCode used in the user’s original project
– allowing the new MSG box to fit in seamlessly without any further
changes to existing code.
INT FUNCTION
DspSIAreYouSure(STRING
sMessage)
INT
ianswer
ianswer
= VbCallReturn(VbCallRun(VbCallOpen("AreYouSureCiVBA")));
RETURN
ianswer
END
Using CiVBA forms allows numerous Msgbox types, as seen by the
following table.
Combinations of these types are used by adding the values together
for those options together, i.e in our example, we has a
“VbOKCancel” type msgbox, with the Second Button as Default
“vbDefaultButton2” the values of these two option add together to
give the 257 used in our CiVBA code.
This list shows the available types
of message boxes.
Constant
|
Value
|
Description
|
vbOKOnly
|
0
|
Display
OK button only.
|
vbOKCancel
|
1
|
Display
OK and Cancel buttons.
|
vbAbortRetryIgnore
|
2
|
Display
Abort, Retry, and Ignore buttons.
|
vbYesNoCancel
|
3
|
Display
Yes, No, and Cancel buttons.
|
vbYesNo
|
4
|
Display
Yes and No buttons.
|
vbRetryCancel
|
5
|
Display
Retry and Cancel buttons.
|
vbCritical
|
16
|
Display
Critical Message icon.
|
vbQuestion
|
32
|
Display Warning
Query icon.
|
vbExclamation
|
48
|
Display Warning
Message icon.
|
vbInformation
|
64
|
Display
Information Message icon.
|
vbDefaultButton1
|
0
|
First button is
default.
|
vbDefaultButton2
|
256
|
Second button
is default.
|
vbDefaultButton3
|
512
|
Third button is
default.
|
vbDefaultButton4
|
768
|
Fourth button
is default.
|
vbApplicationModal
|
0
|
Application
modal; the user must respond to the message box before continuing
work in the current application.
|
vbSystemModal
|
4096
|
System modal;
all applications are suspended until the user responds to the
message box.
|
The values returned
when a particular button is pressed is defined below:
Constant
|
Value
|
Button
|
vbOK
|
1
|
OK
|
vbCancel
|
2
|
Cancel
|
vbAbort
|
3
|
Abort
|
vbRetry
|
4
|
Retry
|
vbIgnore
|
5
|
Ignore
|
vbYes
|
6
|
Yes
|
vbNo
|
7
|
No
|
|